For this part of the Hangfire series, I hacked together an application with the help of GitHub Copilot that allows users to compose a message for different cultures and send them to E-Mail addresses. As the focus is on the Hangfire part, most of the code does not follow best practices: there is no view model or persistence model – just domain objects, repositories are used directly in controller methods, no interfaces are used, etc.
Project Structure
- ExampleApp (sln)
- Web (ASP.NET Core MVC application)
- App (Classlib)
The starting point
Message sending works like this: the user clicks the Send button on a message for which he has already defined the multilingual text and the recipients. That sends a post request to the ASP.NET Core app’s SendController.Send action.
using App;
using Microsoft.AspNetCore.Mvc;
namespace Web.Controllers;
public class SendController(MessageSender messageSender, MessageRepository messageRepository) : Controller
{
public IActionResult Index([FromRoute]int mesageId)
{
return View(messageRepository.GetById(mesageId));
}
[HttpPost]
public async Task<IActionResult> Send(int messageId)
{
await messageSender.Send(messageId);
return RedirectToAction("Index", "Home");
}
}
The controller gets a MessageSender injected by the DI container which it calls to send the messages to the recipients. This class is located not in the Web project, but in the App project. If the implementation of the message sending was in the Web Project, we’d need to extract that into a new project that we can then reference from our HangfireServer project we’ll be creating soon.
The code works, and the messages are being sent, but the user experience is awful. After clicking send, our user does not get any feedback at all, other than a spinner in the browser.

Only after all the messages are sent does the page load again, and the user can continue working. We’ll improve this by offloading the actual mail sending process to Hangfire.
Checking the send implementation for fitness
Any Hangfire job should be re-entrant so that in case the Hangfire Server crashes, it can be picked up again after it has restarted. Let’s take a look at the implementation of the MessageSender.Send method.
using System.Collections.Immutable;
using Microsoft.Extensions.Logging;
namespace App;
public class MessageSender(MessageRepository messageRepository, FallbackCultureProvider fallbackCultureProvider, ILogger<MessageSender> logger)
{
public async Task Send(int messageId)
{
var message = messageRepository.GetById(messageId);
if (message == null)
{
throw new ArgumentException("Message not found");
}
foreach(var recipient in message.DeliverTo.ToImmutableList())
{
string textToSend = GetMessageTextForRecipient(message, recipient);
//Simulate calling SendGrid/some SMTP server/…
await Task.Delay(TimeSpan.FromSeconds(Random.Shared.Next(1, 5)));
message.DeliveredTo.Add(recipient);
message.DeliverTo.Remove(recipient);
messageRepository.SaveMessage(message);
logger.LogInformation($"Message sent to {recipient.Email} ({recipient.SelectedCulture.Iso2Code}): {textToSend}");
}
}
private string GetMessageTextForRecipient(Message message, Receipient recipient)
{
Culture currentCulture = recipient.SelectedCulture;
while(!message.Text.ContainsKey(currentCulture))
{
currentCulture = fallbackCultureProvider.GetFallbackCultureFor(currentCulture);
}
return message.Text[currentCulture];
}
}
The code retrieves the message by ID, creates a snapshot of the recipients it needs to be sent to (DeliverTo), iterates over them one by one, sends the mail and moves the recipient to the DeliveredTo Set. It then persists the altered message object after every mail sent.
What would happen if the Hangfire server stopped processing the job after half of the messages were sent? Let’s say between these two lines:
message.DeliveredTo.Add(recipient);
/*### hanfire dies here ###*/
message.DeliverTo.Remove(recipient);
When Hangfire picks up again, it will invoke the method again from the start, so it will re-load the message from the data store, which looks like this:
DeliveredTo | Ryan, Lucas |
DeliverTo | Nancy, Thomas |
It died after sending the mail to Nancy but before persisting the movement of Nancy from DeliverTo -> DeliveredTo. So Nancy will get the message twice, but Ryan and Lucas won’t. Thomas will get the message too. So, besides some recipient getting a message twice, the code will work fine when interrupted -> it is re-entrant (enough).
Also, the Parameter the Method takes should be a simple value because it needs to be serialized by Hangfire. It’s an int, so that’s fine also.
Adding Hangfire client and dashboard to our Web application
We’ll use the Hangfire metapackage here and use the newer SqlClient Package (Microsoft, not System.).
dotnet add .\Web\ package Hangfire
dotnet add .\Web\ package Microsoft.Data.SqlClient
Now we’ll need to edit the Program.cs file
//…
/*add this*/
builder.Services.AddHangfire(configuration => configuration
.UseSqlServerStorage(builder.Configuration.GetConnectionString("HangfireConnection"))
);
//…
app.MapStaticAssets();
app.MapHangfireDashboard(); /*and this line*/
app.MapControllerRoute(…);
//…
And also add a connection string in appsettings.Development.json to specify the database we want Hangfire to use:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"ConnectionStrings": {
"HangfireConnection": "Server=.;Database=MessagingApp_Hangfire;TrustServerCertificate=true;Integrated Security=true;"
}
}
Now, IBackgroundJobClient can be injected into our Send controller to offload the work to Hangfire Server:
using App;
using Hangfire;
using Microsoft.AspNetCore.Mvc;
namespace Web.Controllers;
public class SendController(IBackgroundJobClient backgroundJobClient, MessageRepository messageRepository) : Controller
{
public IActionResult Index([FromRoute]int mesageId)
{
return View(messageRepository.GetById(mesageId));
}
[HttpPost]
public IActionResult Send(int messageId)
{
backgroundJobClient.Enqueue<MessageSender>(messageSender => messageSender.Send(messageId));
return RedirectToAction("Index", "Home");
}
}
Notice how we no longer need to inject an instance of MessageSender into our Controller. Also, the Send method does not need to be async anymore because we don’t call MessageSender.Send directly. We now just enqueue a job and return immediately.
When we start the application and navigate to /hangfire, we see the Hangfire dashboard, hosted within our web application. After clicking send on a message again, the dashboard shows it was enqueued successfully:
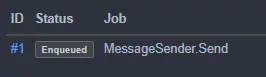
It won’t be processed however, because no server is present at the moment.

Adding Hangfire server
We’ll now create a new console application to host our Hangfire Server and add the necessary packages for Hangfire to work.
dotnet new console -o HangfireServer
dotnet add .\HangfireServer\ package Hangfire.Core
dotnet add .\HangfireServer\ package Hangfire.SqlServer
dotnet add .\HangfireServer\ package Microsoft.Data.SqlClient
If we now add some super simple code into the Program.cs of our new console project and run it what happens?
using Hangfire;
GlobalConfiguration.Configuration
.UseSqlServerStorage("Server=.;Database=MessagingApp_Hangfire;TrustServerCertificate=true;Integrated Security=true;");
using (var _ = new BackgroundJobServer())
{
Console.WriteLine("Hangfire Server started. Press any key to exit…");
Console.ReadKey();
}
Nothing much in the console app. After displaying Hangfire Server started. Press any key to exit… there’s just silence. Our web-app no longer displays the spinner for a long time – great! What does the Hangfire Dashboard in our Web App say?
Failed
Can not change the state to 'Enqueued': target method was not found.
System.IO.FileNotFoundException (<Server>)
Could not resolve assembly 'App'.
We forgot to reference our App assembly, which contains the code we want Hangfire to run on the server. Let’s add that real quick.
dotnet add .\HangfireServer\ reference .\App\
When trying again, we get a different error now:
Failed
System.MissingMethodException (<Server>)
System.MissingMethodException: Cannot dynamically create an instance of type 'App.MessageSender'. Reason: No parameterless constructor defined.
Hangfire cannot create an instance of our MessageSender class because it has no parameterless constructor. Adding one does not help us either because we won’t get our dependencies then:
public class MessageSender(MessageRepository messageRepository, FallbackCultureProvider fallbackCultureProvider, ILogger<MessageSender> logger)
{
//…
}
While we registered our MessageRepository, FallbackCultureProvider and MessageSender in the Web application and the WebApplicationHostBuilder registered a logger factory for us, we have none of that in our HangfireServer project. We don’t even have a dependency injection container.
While multiple extensions exist that work with various DI containers (https://www.hangfire.io/extensions.html#ioc-containers), I prefer to keep things the same as much as possible, so I want to use the Microsoft.Extensions.Hosting package. This also comes with a logger factory bundled, so I don’t have to manually fiddle with the logging infrastructure. It will work just like in the Web application. This will also help in the future in case I want to move more functionality from the Webapp to Hangfire. We also need to install the Hangfire.NetCore package which wires up the JobActivator with the DI container provided by Microsoft.Extensions.Hosting.
dotnet add .\HangfireServer\ package Microsoft.Extensions.Hosting
dotnet add .\HangfireServer\ package Hangfire.NetCore
The Program.cs of our HangfireServer Project is super short. We just register the dependencies our background jobs need (from the App project) and configure Hangfire.
using App;
using Hangfire;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
Host.CreateDefaultBuilder(args)
.ConfigureServices((hostContext, services) =>
{
services.AddSingleton(_ => new MessageRepository( /* … */ ));
services.AddTransient<MessageSender>();
services.AddTransient<FallbackCultureProvider>();
services.AddHangfire(config =>
config.UseSqlServerStorage("Server=.;Database=MessagingApp_Hangfire;TrustServerCertificate=true;Integrated Security=true;"));
services.AddHangfireServer();
})
.Build()
.Run();
And that’s it! When we take a look at the dashboard again we now see the job gets executed successfully.
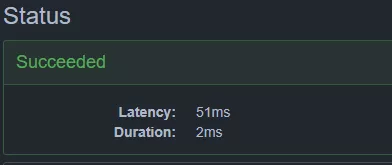
Photo by Mark Hindle on Unsplash